Setting Up Protected Pages
Protected pages are essential for granting users access to your product once they become paying customers. For a user to access these protected pages, they must be signed in and have an active plan.
How to Determine if a User Has an Active Plan
We use a credits system to assess if a user has an active plan. If a user's credits are higher than 0, they will have access to the product. This approach offers several advantages:
- By linking access to the credit balance, you can create products that consume credits. Examples include AI products that use credits as users generate content or games where users can play a limited number of times before losing access.
- For a standard product that grants access upon payment and revokes access when payment is not made, you can manage this by setting credits to 100 or 0.
- All these logics are handled by our payment systems, which currently support Stripe and LemonSqueezy for payments.
How Protected Pages Work
We use a layout specifically for protected routes. This layout verifies user authentication and credit balance before rendering its children. All routes nested under /private
are protected. To change the base protected route, simply rename the "private" folder to your desired name (e.g., "dashboard", "protected", "product").
Detailed Instructions
For more detailed instructions, refer to the comments inside the layout located at app/private/layout.js
.
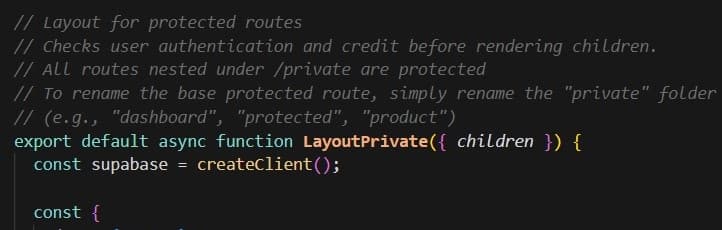
NoCredits Component
Currently, if a user has insufficient credits, we render the NoCredits
component. As an alternative, you can consider redirecting the user to a dedicated page using redirect('/url')
.
Here is the component displayed when a user has insufficient credits, prompting them to purchase a plan. Feel free to enhance this component as needed:
const NoCredits = () => (
<>
<Navbar navigation={null} cta={null} />
<main>
<PricingTable
heading="You do not have an active plan."
description="Please purchase a plan to continue using our services."
/>
</main>
</>
);